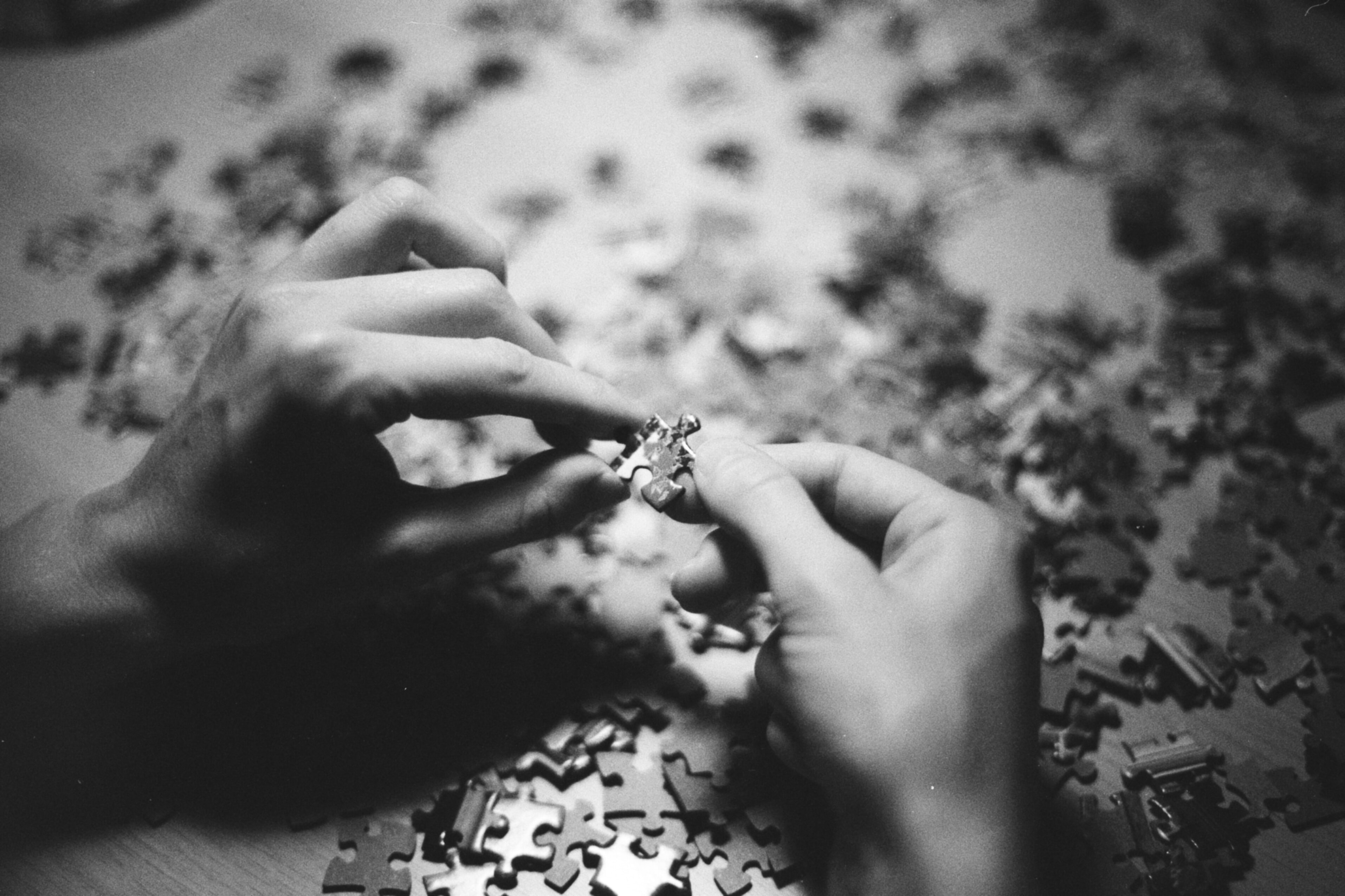
OJ Solution Templates
~3 min read
Solving programming problems is a great way to improve your problem-solving and critical thinking skills. There are several online automated judge platforms where you can submit your code solution to a programming problem and receive a verdict for your submitted solution. Some of the online automated judge platforms are:
In most of the platforms, you have to code the entire solution - starting from taking the input, processing the input, and then providing the output. However, there may be occasions where you solely want to focus on deriving the solution from the input. For such circumstances, I have created OJ Solution Templates, a repository containing solution templates in Java for problems of various online automated judges.
So, suppose you want to solve the programming problem - beecrowd 1001. Extremely Basic. You just need to grab the specific solution template from the repository.
Solution Template
package io.github.tahanima.beecrowd._1001;
import java.util.Scanner;
/**
* @author tahanima
*/
public class Main {
/**
* @param a denotes the first number
* @param b denotes the second number
* @return a string containing the answer
*/
public static String solve(int a, int b) {
// Implement this method
return "";
}
/**
* Takes care of the problem's input and output.
*/
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println(solve(scanner.nextInt(), scanner.nextInt()));
}
}
And then, you need to complete the solve
method. While submitting the solution to the online judge platform, make sure to remove the package definition. Also, feel free to remove the comments.
Submitted Solution
import java.util.Scanner;
public class Main {
public static String solve(int a, int b) {
return String.format("X = %d", a + b);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println(solve(scanner.nextInt(), scanner.nextInt()));
}
}
I hope this initiative turns out helpful for you. Happy Coding 🎉
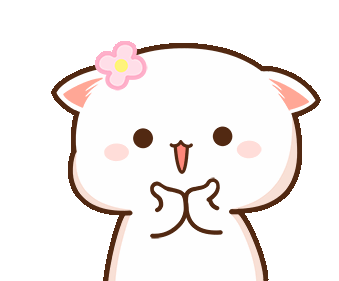